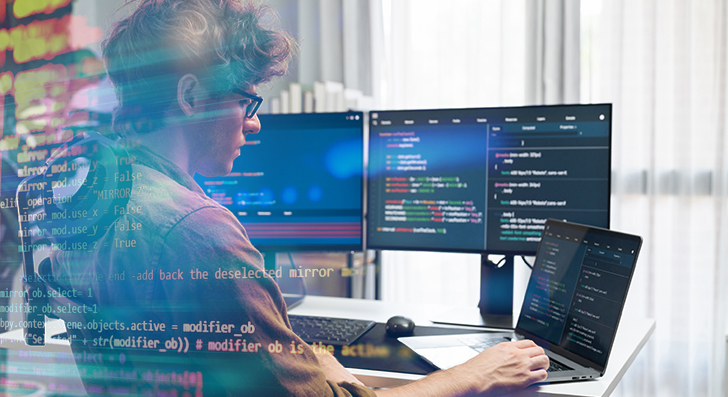
Scalability indicates your application can deal with growth—extra end users, a lot more data, and more targeted visitors—with no breaking. As being a developer, building with scalability in your mind saves time and strain later on. Here’s a transparent and sensible guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be aspect of your respective strategy from the start. Numerous apps fail if they develop rapid since the first layout can’t deal with the additional load. As a developer, you must think early about how your process will behave under pressure.
Start off by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Instead, use modular layout or microservices. These styles crack your application into lesser, independent areas. Each individual module or services can scale on its own devoid of influencing the whole program.
Also, think about your database from day just one. Will it have to have to handle a million consumers or merely 100? Pick the proper form—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them still.
A further important position is to stop hardcoding assumptions. Don’t generate code that only is effective under current circumstances. Take into consideration what would take place Should your consumer foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style patterns that assistance scaling, like message queues or occasion-driven systems. These aid your app deal with much more requests with out getting overloaded.
When you build with scalability in your mind, you are not just getting ready for achievement—you are lowering long term complications. A perfectly-prepared procedure is less complicated to take care of, adapt, and increase. It’s much better to prepare early than to rebuild afterwards.
Use the appropriate Database
Choosing the right databases is usually a critical Section of developing scalable programs. Not all databases are constructed exactly the same, and using the Completely wrong you can sluggish you down as well as result in failures as your app grows.
Get started by comprehending your information. Is it highly structured, like rows inside of a desk? If Indeed, a relational database like PostgreSQL or MySQL is an effective suit. These are generally robust with interactions, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle additional site visitors and details.
When your knowledge is a lot more versatile—like person action logs, products catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and can scale horizontally far more easily.
Also, take into account your study and publish styles. Have you been executing plenty of reads with less writes? Use caching and skim replicas. Have you been managing a heavy compose load? Check into databases that may manage significant write throughput, and even function-centered info storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Consider in advance. You might not need Sophisticated scaling functions now, but picking a databases that supports them indicates you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data based on your access patterns. And usually watch databases overall performance as you expand.
In a nutshell, the best database is determined by your app’s construction, speed desires, And just how you be expecting it to improve. Acquire time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is key to scalability. As your application grows, just about every smaller delay adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your technique. That’s why it’s vital that you Develop efficient logic from the start.
Get started by producing cleanse, straightforward code. Steer clear of repeating logic and take away nearly anything unneeded. Don’t choose the most elaborate Option if a simple a single functions. Keep the features short, concentrated, and simple to check. Use profiling equipment to locate bottlenecks—sites wherever your code will take too long to operate or utilizes far too much memory.
Following, look at your databases queries. These often sluggish issues down in excess of the code itself. Ensure that Each and every query only asks for the information you actually need to have. Avoid Pick out *, which fetches every thing, and as a substitute choose distinct fields. Use indexes to hurry up lookups. And keep away from accomplishing too many joins, especially across significant tables.
In the event you detect the same knowledge remaining requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app far more successful.
Make sure to check with massive datasets. Code and queries that do the job fine with 100 information may well crash if they have to take care of one million.
To put it briefly, scalable apps are quick apps. Keep your code restricted, your queries lean, and use caching when wanted. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more customers and much more site visitors. If anything goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors across various servers. In lieu of a person server executing the many operate, the load balancer routes consumers to distinct servers according to availability. This means no one server will get overloaded. If a single server goes down, the load balancer can send visitors to the others. Applications like Nginx, HAProxy, or cloud-based mostly answers from AWS and Google Cloud make this easy to build.
Caching is about storing knowledge temporarily so it might be reused promptly. When consumers request the exact same data again—like an item webpage or possibly a profile—you don’t have to fetch it within the database each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files close to the person.
Caching minimizes databases load, improves pace, and makes your app extra productive.
Use caching for things which don’t alter often. And constantly make sure your cache is up-to-date when details does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your app manage additional users, remain rapid, and Recuperate from complications. If you plan to expand, you would like each.
Use Cloud and Container Equipment
To develop scalable applications, you'll need equipment that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you overall flexibility, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit read more you to hire servers and products and services as you'll need them. You don’t need to acquire hardware or guess foreseeable future ability. When website traffic improves, you could include a lot more assets with just a couple clicks or routinely employing car-scaling. When targeted traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to creating your app rather than managing infrastructure.
Containers are Yet another important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into a person device. This makes it easy to maneuver your application among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Instrument for this.
Once your application makes use of multiple containers, applications like Kubernetes make it easier to control them. Kubernetes handles deployment, scaling, and recovery. If just one element of your application crashes, it restarts it instantly.
Containers also make it straightforward to independent parts of your application into solutions. You could update or scale pieces independently, that's great for effectiveness and reliability.
Briefly, making use of cloud and container applications signifies you can scale rapid, deploy effortlessly, and Get well rapidly when challenges occur. If you prefer your app to improve with out boundaries, start applying these equipment early. They help you save time, decrease possibility, and assist you to keep centered on developing, not repairing.
Observe Every thing
In case you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, place issues early, and make far better selections as your application grows. It’s a key A part of constructing scalable devices.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—keep track of your app also. Regulate how much time it's going to take for users to load pages, how often errors occur, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes above a Restrict or simply a company goes down, you'll want to get notified promptly. This helps you take care of challenges rapid, generally ahead of consumers even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you are able to roll it again ahead of it triggers real destruction.
As your app grows, visitors and details raise. Devoid of monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the proper applications in position, you continue to be on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring it really works effectively, even stressed.
Ultimate Views
Scalability isn’t just for major corporations. Even little applications require a robust foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without breaking under pressure. Start out little, Consider significant, and Construct clever.